IDE Integration
File Opener Preference
When clicking on a file path from the Test Runner in the command log or an error, Cypress will attempt to open the file on your system. If the editor supports inline highlighting of the file, the file will open with the cursor located on the line and column of interest.
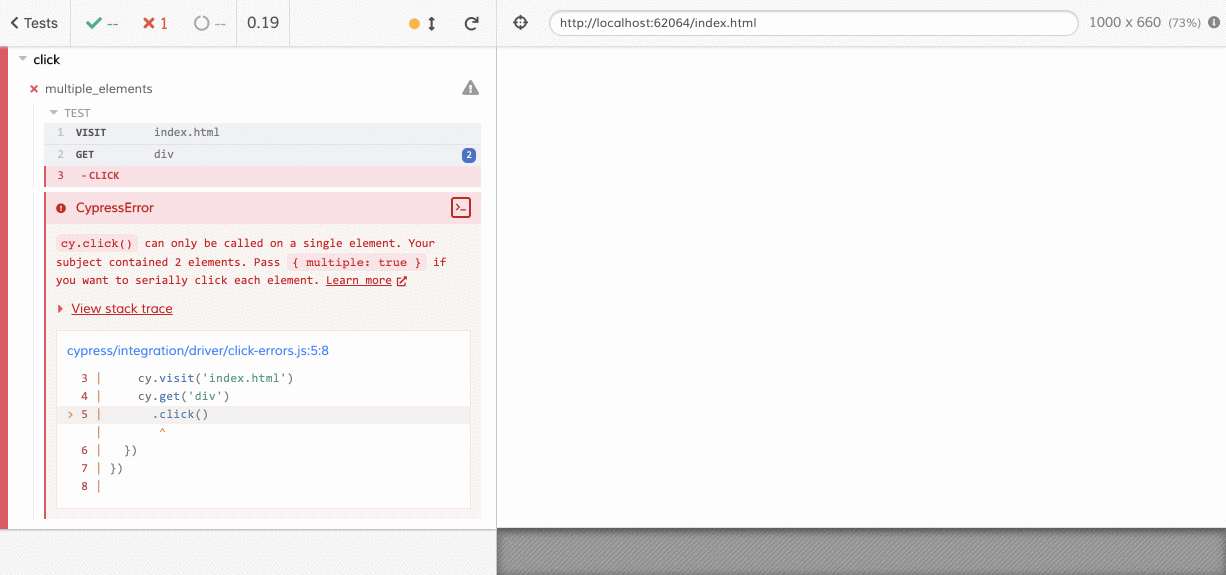
The first time you click a file path, Cypress will prompt you to select which location you prefer to open the file. You can choose to open it in your:
- File system (e.g. Finder on MacOS, File Explore on Windows)
- An IDE located on your system
- A specified application path
Cypress attempts to find available file editors on your system and display those as options. If your preferred editor is not listed, you can specify the (full) path to it by selecting Other. Cypress will make every effort to open the file, but it is not guaranteed to work with every application.
After setting your file opener preference, any files will automatically open in your selected application without prompting you to choose. If you want to change your selection, you can do so in the Settings tab of the Cypress Test Runner by clicking under File Opener Preference.
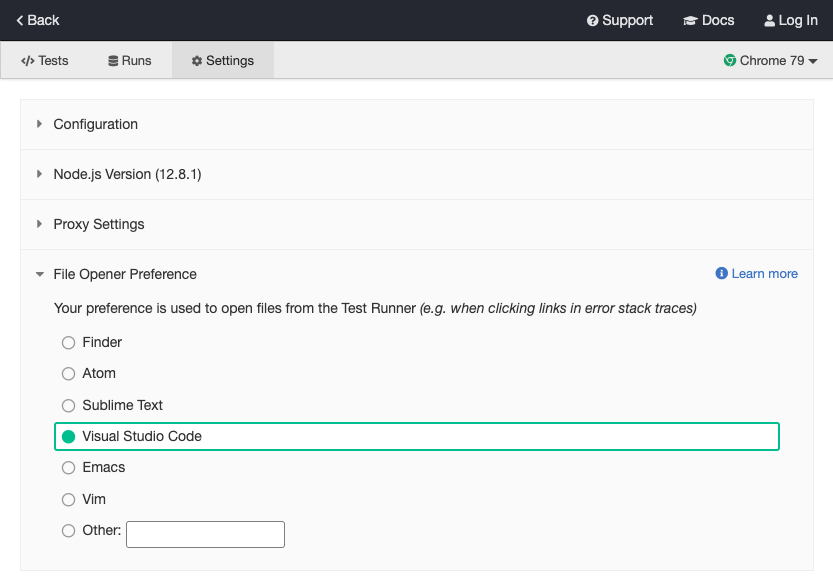
Extensions & Plugins
There are many third-party IDE extensions and plugins to help integrate your IDE with Cypress.
Visual Studio Code
- Cypress Fixture-IntelliSense:
Supports your cy.fixture() and
cy.route(..., "fixture:")
commands by providing intellisense for existing fixtures. - Cypress Helper: Various helpers and commands for integration with Cypress.
- Cypress Snippets: Useful Cypress code snippets.
- Cypress Snippets: This extension includes the newest and most common cypress snippets.
- Open Cypress:
Allows you to open Cypress specs and single
it()
blocks directly from VS Code. - Test Utils:
Easily add or remove
.only
and.skip
modifiers with keyboard shortcuts or the command palette.
IntelliJ Platform
Compatible with IntelliJ IDEA, AppCode, CLion, GoLand, PhpStorm, PyCharm, Rider, RubyMine, and WebStorm.
- Cypress Support: Integrates Cypress under the common Intellij test framework.
- Cypress Support Pro: An improved version of Cypress Support plugin with debugging from the IDE, advanced autocomplete, built-in recorder and other features.
Intelligent Code Completion
Writing Tests
Features
IntelliSense is available for Cypress. It offers intelligent code suggestions directly in your IDE while writing tests. A typical IntelliSense popup shows command definition, a code example and a link to the full documentation page.
Autocomplete while typing Cypress commands
Signature help when writing and hovering on Cypress commands
Autocomplete while typing assertion chains, including only showing DOM assertions if testing on a DOM element.
Set up in your Dev Environment
This document assumes you have installed Cypress.
Cypress comes with TypeScript type declarations included. Modern text editors can use these type declarations to show IntelliSense inside spec files.
Triple slash directives
The simplest way to see IntelliSense when typing a Cypress command or assertion is to add a triple-slash directive to the head of your JavaScript or TypeScript testing file. This will turn the IntelliSense on a per file basis. Copy the comment line below and paste it into your spec file.
/// <reference types="Cypress" />
If you write custom commands and provide
TypeScript definitions for them, you can use the triple slash directives to show
IntelliSense, even if your project uses only JavaScript. For example, if your
custom commands are written in cypress/support/commands.js
and you describe
them in cypress/support/index.d.ts
use:
// type definitions for Cypress object "cy"
/// <reference types="cypress" />
// type definitions for custom commands like "createDefaultTodos"
/// <reference types="../support" />
See the
cypress-example-todomvc
repository for a working example.
If the triple slash directive does not work, please refer to your code editor in TypeScript's Editor Support doc and follow the instructions for your IDE to get TypeScript support and intelligent code completion configured in your developer environment first. TypeScript support is built in for Visual Studio Code, Visual Studio, and WebStorm - all other editors require extra setup.
jsconfig
Reference type declarations via Instead of adding triple slash directives to each JavaScript spec file, some
IDEs (like VS Code) understand a common jsconfig.json
file in the root of the
project. In that file, you can include the Cypress module and your test folders.
{
"include": ["./node_modules/cypress", "cypress/**/*.js"]
}
The Intelligent Code Completion should now show help for cy
commands inside
regular JavaScript spec files.
tsconfig
Reference type declarations via Adding a
tsconfig.json
inside your
cypress
folder
with the following configuration should get intelligent code completion working.
{
"compilerOptions": {
"allowJs": true,
"types": ["cypress"]
},
"include": ["**/*.*"]
}
Configuration
Features:
When editing the
configuration file (cypress.json
by default),
you can use our json schema file to
get intelligent tooltips in your IDE for each configuration property.
Property help when writing and hovering on configuration keys
Properties list with intelligent defaults
Set up in your Dev Environment:
Intelligent code completion using JSON schemas is supported by default in Visual Studio Code and Visual Studio. All other editors will require extra configuration or plugins for JSON schema support.
To set up in Visual Studio Code you can open
Preferences / Settings / User Settings
and add the json.schemas
property.
Make sure to replace cypress.json
with your configuration file if not the
default.
{
"json.schemas": [
{
"fileMatch": ["cypress.json"],
"url": "https://on.cypress.io/cypress.schema.json"
}
]
}
Or you can directly add a $schema
key to your Cypress configuration file,
which is a great way to share the schema with all collaborators of the project.
"$schema": "https://on.cypress.io/cypress.schema.json",